Today, I will explain few things that I've personally had lots of difficulties, at first, understanding and then while applying.
As all the three things that I'll be discussing are delegates, let's first see what exactly a delegate is.
"When a Head of State dies, the President of the United States sometimes does not have time to attend the funeral personally. Instead, he dispatches a delegate. Often this delegate is the Vice President, but sometimes the VP is unavailable and the President must send someone else, such as the Secretary of State or even the First Lady. He does not want to 'hardwire' his delegated authority to a single person; he might delegate this responsibility to anyone who is able to execute the correct international protocol.
The President defines in advance what responsibility will be delegated(attend the funeral), what items will be passed(condolences, kind words), and what value he hopes to get back(good will). He then assigns a particular person to that delegated responsibility at "runtime" as the course of his presidency progresses."
Delegates in our programming world also behave in somewhat similar fashion.
MSDN talks about delegate as follows:-
"A delegate is a reference type that can be used to encapsulate a named or an anonymous method. Delegates are similar to function pointers in C++; however, delegates are type-safe and secure."
So, a delegate is a reference type, as opposed to a value type, which is used to encapsulate a named or an anonymous method. As we know, an anonymous method is nothing but a method for which we have not provided any name and is provided by the compiler itself.
The most common example that we have for delegates are event handlers. In ASP.NET WebForms, we create a control and bind event handlers for various of its events. Here, effectively, we are just binding the event handler methods.
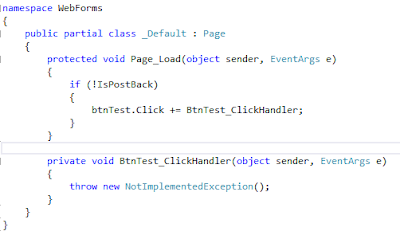
As we can see in the picture above, after registration, this BtnTest_ClickHandler sits there calmly and quietly, doing nothing until the event is triggered. As soon as the event is triggered, the click handler goes and executes the code inside itself.
So, if you want to perform some action at some later point-in-time or after some event, go ahead and create a delegate. Now, create a method which takes care of your action. This method needs to have the same signature as that of the delegate. Then, just wire up the two(delegate and method). That's all. Whenever you need to perform that action, just invoke the delegate with appropriate parameters and the target method will be invoked.
The most common scenario where we use Func is when we are filtering a list using Where condition.

Here, since my list is of type int, Where expects int as the input and will return a bool as the output.
The most common scenario where we use Action is an event handler. We provide the method with parameters such as the sender and EventArgs but the method does not return any value(as shown in the first image above).
So, effectively, Predicate is just a Func<T, bool>.
Some of the functions inside List expect Predicates as a parameter to be passed to them, as shown below.
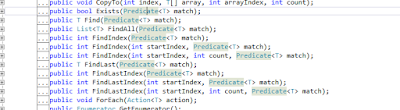
The flowchart below should help you decide when to choose which delegate even better.
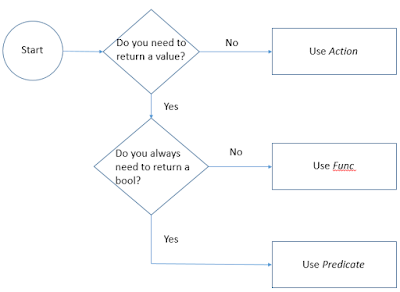
So, that's all there is to these confusing terms.
Hope this helps.
As all the three things that I'll be discussing are delegates, let's first see what exactly a delegate is.
What is a DELEGATE?
To understand delegates, I got this wonderful real-world scenario stated here, which I'm quoting below."When a Head of State dies, the President of the United States sometimes does not have time to attend the funeral personally. Instead, he dispatches a delegate. Often this delegate is the Vice President, but sometimes the VP is unavailable and the President must send someone else, such as the Secretary of State or even the First Lady. He does not want to 'hardwire' his delegated authority to a single person; he might delegate this responsibility to anyone who is able to execute the correct international protocol.
The President defines in advance what responsibility will be delegated(attend the funeral), what items will be passed(condolences, kind words), and what value he hopes to get back(good will). He then assigns a particular person to that delegated responsibility at "runtime" as the course of his presidency progresses."
Delegates in our programming world also behave in somewhat similar fashion.
MSDN talks about delegate as follows:-
"A delegate is a reference type that can be used to encapsulate a named or an anonymous method. Delegates are similar to function pointers in C++; however, delegates are type-safe and secure."
So, a delegate is a reference type, as opposed to a value type, which is used to encapsulate a named or an anonymous method. As we know, an anonymous method is nothing but a method for which we have not provided any name and is provided by the compiler itself.
The most common example that we have for delegates are event handlers. In ASP.NET WebForms, we create a control and bind event handlers for various of its events. Here, effectively, we are just binding the event handler methods.
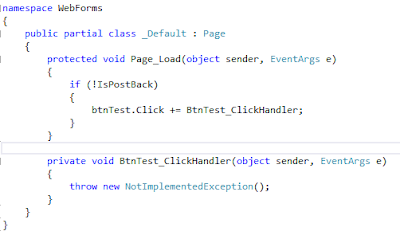
As we can see in the picture above, after registration, this BtnTest_ClickHandler sits there calmly and quietly, doing nothing until the event is triggered. As soon as the event is triggered, the click handler goes and executes the code inside itself.
So, if you want to perform some action at some later point-in-time or after some event, go ahead and create a delegate. Now, create a method which takes care of your action. This method needs to have the same signature as that of the delegate. Then, just wire up the two(delegate and method). That's all. Whenever you need to perform that action, just invoke the delegate with appropriate parameters and the target method will be invoked.
Func
Func is just a parameterised delegate which accepts zero or more inputs but definitely returns an output. So, effectively, if you have a scenario where you want to return an output, go for Func.The most common scenario where we use Func is when we are filtering a list using Where condition.

Here, since my list is of type int, Where expects int as the input and will return a bool as the output.
Action
Action is also a parameterised delegate which accepts zero or more inputs but does not return an output. So, if you don't want to return an output, go for Action.The most common scenario where we use Action is an event handler. We provide the method with parameters such as the sender and EventArgs but the method does not return any value(as shown in the first image above).
Predicate
Predicate is nothing but a specialized Func where the return type is always a bool.So, effectively, Predicate is just a Func<T, bool>.
Some of the functions inside List expect Predicates as a parameter to be passed to them, as shown below.
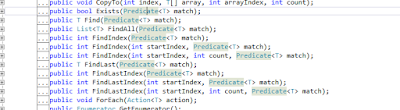
The flowchart below should help you decide when to choose which delegate even better.
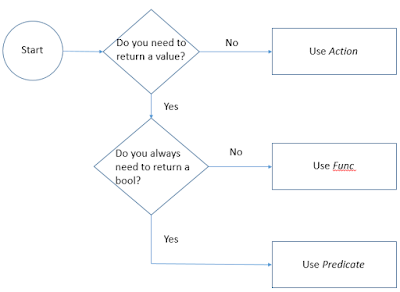
So, that's all there is to these confusing terms.
Hope this helps.